First create a set of images you want to show for sequence of progress bar.
In this example, these were the images taken to show percentages of battert level from 10 to 100.
level-list is a XML definition of a drawable resource which manages alternate drawables for different max & minimum levels. This can be applied to a View, or widget ,ImageView,Button etc. Once this is applied, the levels can be changed using api,
setLevel() and setImageLevel()
1 - Consider the below xml file [ images.xml ]. It defines the maxLevel values and its corresponding drawable ( in this example, it picks up the different battery images] .
images.xml:
-----------
<?xml version="1.0" encoding="utf-8"?>
<level-list xmlns:android="http://schemas.android.com/apk/res/android">
<item android:maxLevel="0" android:drawable="@drawable/batt_10" />
<item android:maxLevel="1" android:drawable="@drawable/batt_20" />
<item android:maxLevel="2" android:drawable="@drawable/batt_30" />
<item android:maxLevel="3" android:drawable="@drawable/batt_40" />
<item android:maxLevel="4" android:drawable="@drawable/batt_50" />
<item android:maxLevel="5" android:drawable="@drawable/batt_60" />
<item android:maxLevel="6" android:drawable="@drawable/batt_70" />
<item android:maxLevel="7" android:drawable="@drawable/batt_80" />
<item android:maxLevel="8" android:drawable="@drawable/batt_90" />
<item android:maxLevel="9" android:drawable="@drawable/batt_100" />
</level-list>
2 - Create a imageView in main.xml which forms the activities content. And note here, set the images.xml as a src to imageView property.
android:src="@drawable/images"
Layout:
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="battery-progress"
/>
<ImageView
android:id="@+id/battery"
android:layout_width="fill_parent"
android:layout_height="40px"
android:src="@drawable/images"
/>
</LinearLayout>
3 - Inside the activity we are gonna access the imageView and set a setImagelevel() api with different values from 0 to 10 and repeat this for indefinite number of times.
package com.android.imageselector;
import java.util.Timer;
import java.util.TimerTask;
import android.app.Activity;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.widget.ImageView;
public class imageselector extends Activity {
/** Called when the activity is first created. */
int i=0;
ImageView v;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
v = (ImageView)findViewById(R.id.battery);
int delay = 1000; // delay for 1 sec.
int period = 1000; // repeat every 10 sec.
Timer timer = new Timer();
final Handler messageHandler = new Handler() {
public void handleMessage(Message msg) {
super.handleMessage(msg);
if(msg.what == 0)
{
if(i<10)
{
i++;
v.setImageLevel(i);
}
else
{
i=0;
v.setImageLevel(i);
}
}
}
};
timer.scheduleAtFixedRate(new TimerTask()
{
public void run()
{
messageHandler.sendEmptyMessage(0);
}
}, delay, period);
}
}
4 - A timer object is created and scheduleAtFixedRate() api is used to invoke the thread for every 1 sec and call Handler object to set the imagelevel value from 0 to 10.
5 - Now the total outcome looks like, the battery images are set for values from 0 to 10 from the images.xml file. And looks like a battery is charged.Similarly the images can be changed as per your requirement to show the circular progress bar images, or horizontal progress bar images based on the progress levels.
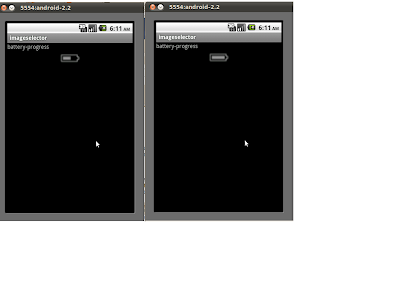
Why dont't you use Handler::sendMessageDelayed() instead of TimerTask.
ReplyDeleteAnd branching at line 32 can be collapsed as i%=10.
its help me lot
ReplyDeletethanks helped me
ReplyDeletegreat example thanks
ReplyDelete